Mon May 08 2023
Array Iteration Part 2
JavaScript111 views

File Name: js-array-iteration2.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Array Iteration in JavaScript: Part 2</title>
<style>
section {
display: flex;
gap: 10;
justify-content: space-between;
}
section div.col {
width: calc(100% / 4);
}
section div.col > div {
margin: 10px 0;
}
button { margin-bottom: 10px; }
</style>
<script>
let num = [25, 45, 81, 64, 10, 46, 89, 49, 22, 40, 78, 62];
const getReduce = () => {
const sum = num.reduce((total, value, index, array) => total+value);
document.getElementById('reduceData').innerHTML = 'Sum From Left Side: ' + sum;
}
const getReduceRight = () => {
const sum = num.reduceRight((total, value, index, array) => total + value);
document.getElementById('rRightData').innerHTML = 'Sum From Left Side: ' + sum;
}
const getEvery = () => {
const allOver = num.every((value) => value > 50);
document.getElementById('everyData').innerHTML = allOver;
}
const getSome = () => {
const isTrue = num.some((value) => value < 80);
document.getElementById('someData').innerHTML = isTrue;
}
</script>
</head>
<body>
<section>
<div class="col">
<button type="button" onclick="getReduce()">Reduce Iteration</button>
<div id="reduceData"></div>
</div>
<div class="col">
<button type="button" onclick="getReduceRight()">Reduce Right Iteration</button>
<div id="rRightData"></div>
</div>
<div class="col">
<button type="button" onclick="getEvery()">Every Iteration</button>
<div id="everyData"></div>
</div>
<div class="col">
<button type="button" onclick="getSome()">Some Iteration</button>
<div id="someData"></div>
</div>
</section>
</body>
</html>
Result Screenshot(s)
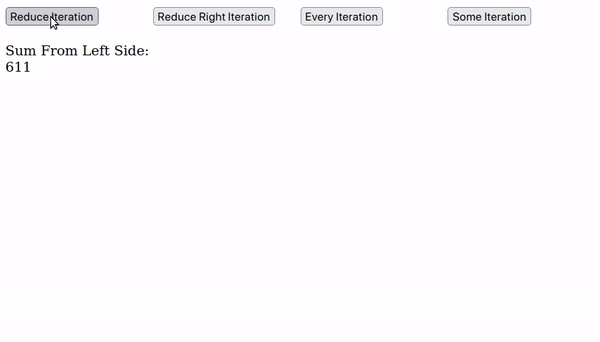
Author:Geekboots