Mon Jul 12 2021
Concept of encapsulation in oops
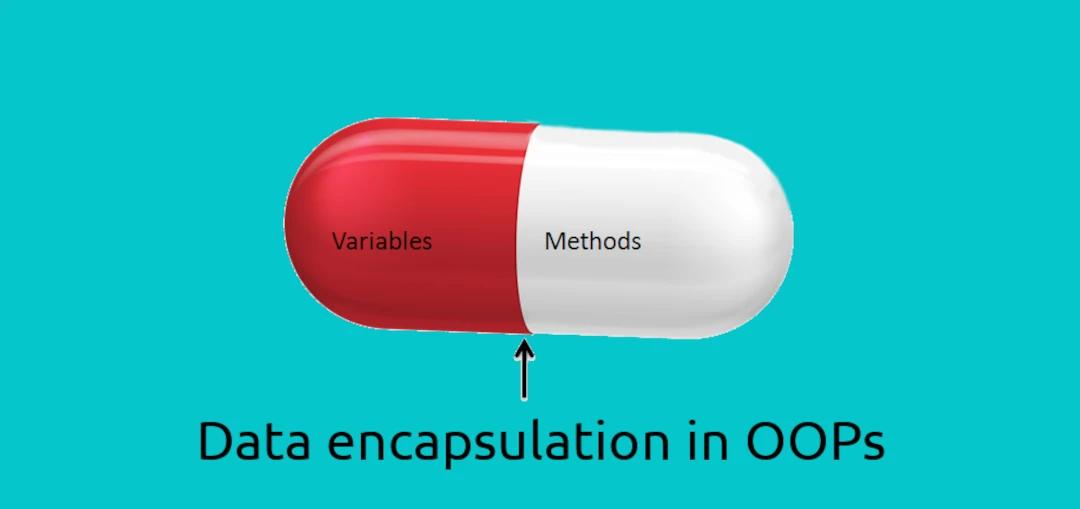
Data security is the biggest concert of the 21st century. To provide basic data protection, encapsulation was implemented in object-oriented programming. Encapsulation is closely related to abstraction and data hiding, in one-word encapsulation is a protective shield. It is one of the fundamentals of OOP which hide data and structure of methods inside a class. It prevents unauthorized access to data and how it processing inside a class.
It is the inclusion within a program object of all the resources needed for the object to properly function on its own - basically, the methods and the data.
Stephen Gilbert and Bill McCarty define encapsulation as “the process of packaging your program, dividing each of its classes into two distinct parts: the interface and the implementation.”
Benefits of using encapsulation
-
By keeping data private and providing public well-defined service methods the role of the object becomes clear to other objects. This increases usability.
-
Other objects are aware of the format to send messages into the object providing the public service. This is essentially a contract between the two objects. The invoker is agreeing to send the message in the specific format, including passing any of the required parameter information. The invoked object is agreeing to process the message and if necessary, return a value in the pre-specified format.
-
The shielding of the data means that should the format of the private data need to change for some reason the objects invoking methods on the object whose data has changed will not need to change the format they send their messages in. If a client object is updating the value directly then by changing the data type you will also need to change the code in the client object. If you are dealing with large systems with hundreds of classes this type of situation can quickly get out of hand with several layers of "knock-on" changes required. Therefore, encapsulation promotes maintenance because code changes can be made independently without affecting other classes.
-
From a software development perspective by having a well defined public services encapsulation allows other developers to quickly understand your code and reuse it in other applications.
-
Encapsulation doesn't mean that you shouldn't expose any data at all. The other important point is to hide the internals of the object to protect its integrity by preventing users from setting the internal data of the object into an invalid or inconsistent state.
-
Importance of encapsulation explained by the need to produce cohesive pieces of code (classes) that solve given problems once and for all so that programmers can use those classes without having to learn about the intricate details of the implementations.
-
Encapsulation helps in isolating implementation details from the behavior exposed to clients of a class and gives you more control over coupling in your code.
-
Encapsulation prevents people who work on your code from making mistakes, by making sure that they only access things they're supposed to access.
-
Encapsulation allows you to formalize your interfaces, separating levels of abstraction.
-
Encapsulation hides unnecessary complexity from other users of the container class.
The types of encapsulation
Data encapsulation
In object oriented programming, data encapsulation is the process that use to hide all the member's data from an outsider and can only access by its own member functions, which can be done by declaring all the member variables as Private.
Example:
class employee {
private:
//private data member
int empId;
public:
//set value
void setEmpId(int id);
//get value
int getEmpId();
}
Method encapsulation
Method encapsulation mainly used to protect your logical implementation from others by declaring them as Private.
Example:
class employee {
private:
//private data member
float salary;
//private method
void calculateDA();
public:
//set value
void setSalary(float amount);
//get DA amount
float getDA();
};
Class encapsulation
Class encapsulation used for internal implementations of your API. If you want to hide the unnecessary class details from others, then you can use class encapsulation.
Example:
class Circle {
private:
//private data member
int diameter;
//private method
void calculateCircumference();
//private class
class Gradient {
public:
gradient();
~gradient();
void setGradient(float uValue);
};
public:
//constructor
circle();
~circle();
//setter function
void setDiameter(float uValue);
//getter function
float getDiameter();
};
Encapsulation in Python
As Python is an object oriented language, you can also protect your methods and variables with the help you of encapsulation. No need to add any private keyword like other language but you can make variable and method private by adding two underscore characters at the beginning of their name.
# Private variable
__maxspeed = 100
# Private method
def __display(self):
print 'Hello world'
Encapsulation in Java
Java support encapsulation mechanism which use to bind codes and data together. On the other way, encapsulation is a protective shield that prevents the data from being accessed by an outsider of that shield. Declaring all the variables in the class as private and set and get data by writing public member function.
public class emp {
private String name; //private variable
// Public methods
public getName(String value) {
name = value;
}
public getName() {
return name;
}
}
Encapsulation in C++
Similar to Java, C++ also use public, private and protected keywords for encapsulation. The member function which manipulates the private data members should be labeled as a public to access them from outside.
class emp {
public:
double getSalary() {
return salary;
}
private:
double salary;
};