Mon Feb 26 2024
Exploring JavaScript Data Types and Their Utility
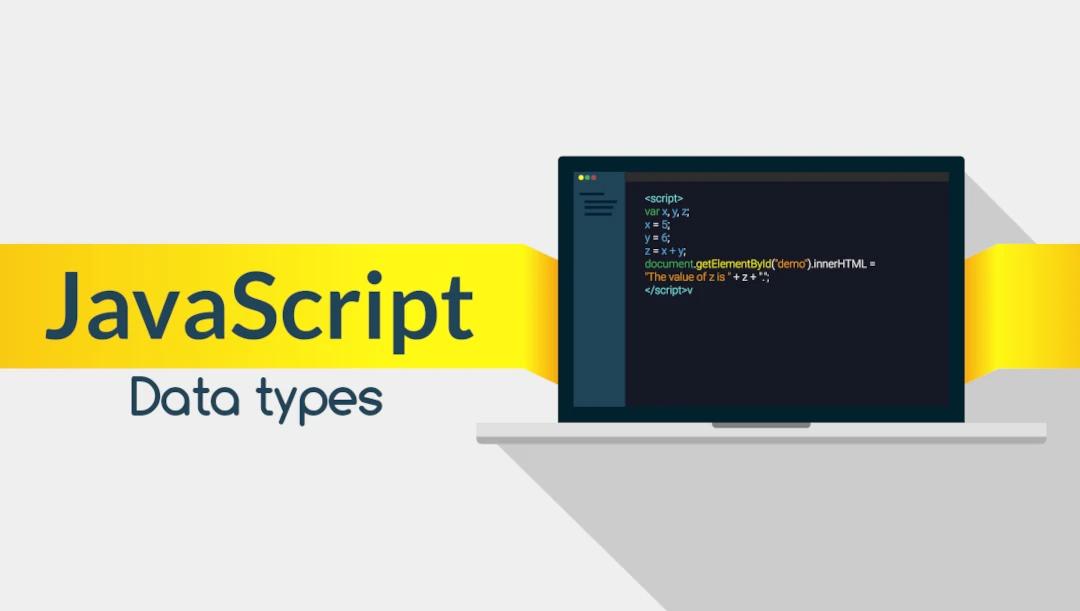
JavaScript, as a dynamically typed language, offers a range of data types to handle different kinds of values and operations. Understanding these data types and their utility is fundamental for every JavaScript developer. In this article, we'll explore the various data types in JavaScript and how they are used.
Primitive Data Types
JavaScript has six primitive data types:
1. Number
Represents numeric values, both integers and floating-point numbers. There are many operations for numbers, e.g. multiplication *, division /, addition +, subtraction - and so on. Besides regular numbers, there are so-called "special numeric values" which also belong to that type: Infinity, -Infinity and NaN (Not-a-Number).
- "Infinity" represents the mathematical Infinity ∞. It is a special value that's greater than any number.
- "NaN" represents a computational error. It is a result of an incorrect or an undefined mathematical operation.
// Number
let age = 30;
2. String
Represents textual data, a series of characters. Strings are written with single ('') or double ("") quotes. You can use quotes inside a string, as long as they don't match the quotes surrounding the string.
// String
let name = "John";
3. Boolean
Represents a logical value, either true or false. Boolean are often used in conditional testing. If the value parameter is omitted or is 0, -0, null, false, NaN, undefined, or the empty string (" "), the object has an initial value of false.
// Boolean
let isAdult = true;
4. Undefined
Represents a variable that has been declared but not assigned a value. The type is also undefined. Any variable can be emptied, by setting the value to undefined. The type will also be undefined.
// Undefined
let city;
5. Null
Represents the intentional absence of any value. It is supposed to be something that doesn't exist. Unfortunately, in JavaScript, the data type of null is an object. You can empty an object by setting it to null.
// Null
let phoneNumber = null;
6. Symbol (ES6)
Represents a unique identifier, introduced in ECMAScript 6. A Symbol is a unique and immutable primitive value and may be used as the key of an Object property. In some programming languages, Symbols are called atoms.
// Symbol
const key = Symbol("unique");
Complex Data Types
JavaScript also has two complex data types:
1. Object
Represents a collection of key-value pairs, where keys are strings and values can be of any data type. JavaScript objects are written with curly braces. Object properties are written as name:value pairs, separated by commas. Objects are the most complex data type.
// Object
let person = { name: "Alice", age: 25 };
2. Function
Represents a callable object that can execute a block of code. It can be treated just like any other data type, including being assigned to variables, passed as arguments to other functions, and returned as values from other functions.
// Function
function greet(name) {
console.log("Hello, " + name + "!");
}
greet("World");
// Assigning a function to a variable
const greet = function(name) {
console.log("Hello, " + name + "!");
};
greet("World");
3. Array
Represents a fixed-size sequential collection of elements of the same type. JavaScript arrays are written with square brackets. Array items are separated by commas. An array is used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type. The Array parameter is a list of strings or integers. When you specify a single numeric parameter with the Array constructor, you specify the initial length of the array. The maximum length allowed for an array is 4,294,967,295.
// String Array
let animal = ['lion', 'tiger', 'elephant'];
// Number Array
let usrId= [12, 20, 25, 30];
4. The 'typeof' operator
The typeof operator is a powerful tool used to determine the data type of a given value.. It's useful when we want to process the values of different types differently, or just want to make a quick check. It returns a string indicating the type of the operand. You can use the JavaScript typeof operator to find the type of JavaScript variable.
typeof undefined; // "undefined"
typeof true; // "boolean"
typeof 42; // "number"
typeof "Hello"; // "string"
typeof BigInt(42); // "bigint"
typeof Symbol(); // "symbol"
typeof function() {}; // "function"
typeof {}; // "object"
typeof []; // "object"
typeof null; // "object"
5. BigInt
With the introduction of BigInt in ECMAScript 2020, JavaScript now supports arbitrarily large integers. BigInts are useful when dealing with operations that involve extremely large integers, such as cryptographic algorithms, mathematical computations, or scenarios where precision is critical. Unlike regular JavaScript numbers, which are limited to 64-bit precision, BigInts can represent integers of arbitrary size.
// BigInt literal
let bigNumber = 9007199254740991n;
Conclusion
Understanding JavaScript data types and their utility is essential for writing effective and efficient code. By leveraging the appropriate data types for different scenarios, developers can create robust and scalable applications that meet the diverse requirements of modern web development. Whether it's storing numeric values, processing text, or defining complex data structures, JavaScript offers a versatile set of data types to handle a wide range of tasks.